Google has added support for in-app updates, this is a new feature introduced in Play Core Library 1.5.0 (as of now is in 1.9.0).
Here is how can be implemented summarily and now we will see a very minimal practical example of Immediate update.
public static void checkUpdates(AppCompatActivity ctx) {
AppUpdateManager appUpdateManager = AppUpdateManagerFactory.create(ctx);
Task<AppUpdateInfo> appUpdateInfoTask = appUpdateManager.getAppUpdateInfo();
appUpdateInfoTask.addOnSuccessListener(appUpdateInfo -> {
if (appUpdateInfo.updateAvailability() == UpdateAvailability.UPDATE_AVAILABLE && appUpdateInfo.isUpdateTypeAllowed(AppUpdateType.IMMEDIATE)) {
// Request the update.
try {
appUpdateManager.startUpdateFlowForResult(
// Pass the intent that is returned by 'getAppUpdateInfo()'.
appUpdateInfo,
AppUpdateType.IMMEDIATE,
// The current activity making the update request.
ctx,
// Include a request code to later monitor this update request.
MY_REQUEST_CODE);
appUpdateManager.registerListener(state -> {
if (state.installStatus() == InstallStatus.DOWNLOADED)
appUpdateManager.completeUpdate();
});
} catch (IntentSender.SendIntentException e) {
Log.e("myapp", "checkUpdates: Error in the update intent: ", e);
}
}
});
appUpdateInfoTask.addOnFailureListener(e -> Log.e("myapp", "checkUpdates 2: Error in the update intent:", e));
}
This will launch a full-screen window intent with a Google-Play-style app update
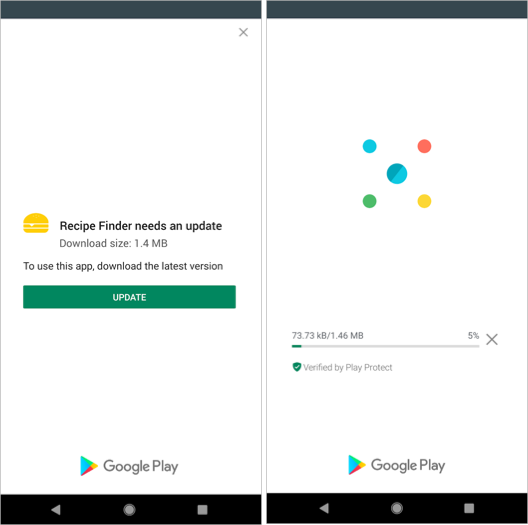
You can add the call to this static method any time in you app, I recommend to put it in a splash screen o during the loading of the main activity.